Custom Keyboards in iOS
How to set up a custom keyboard (input view) for a text field in iOS.
So you want to make a custom keyboard in your iOS app? Numeric, different key positions, custom button sizing – the possibilities are endless!
The tl;dr version: check out the sample code on GitHub.
High-level view
When a text field becomes the first responder, iOS will show the keyboard view on screen. If it isn’t already there, it usually slides up from the bottom.
What you want to do is replace the usual keyboard with your own custom view.
A view is a view
Your custom keyboard view is just that, a view. You can construct it using code or from a nib.
Let’s say you want to build the ultimate programmer nerd’s keyboard, something like this:
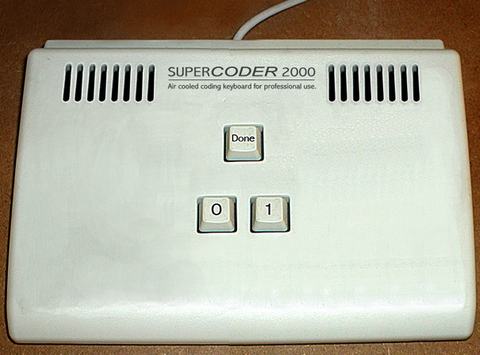
Here’s what a nib-ified version might look like:
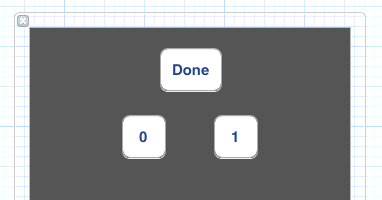
Connecting the keyboard cables
You have your text field, and you have your custom keyboard nib. Connecting them together is easy!
NerdKeyboard *nerdKeyboard = [[NerdKeyboard alloc] initWithNibName:@"NerdKeyboard"
bundle:nil];
[self.myTextField setInputView:nerdKeyboard.view];
Easy! At this point, things will look pretty good. The keyboard appears as expected, but nothing happens when you tap the buttons. Hey, it’s a custom keyboard, remember? It’s up to you to handle that part.
In the sample project, the nib has an accompanying view controller class to handle the button press actions and update the text in the text field. Check out NerdKeyboard.m for the details.
Keyboard clicks
One more small detail: there are no keyboard click sounds! The magic method call you need looks like this:
[[UIDevice currentDevice] playInputClick];
Unfortunately, it’s not that simple. You can’t call playInputClick
anywhere you please; iOS limits its use to very specific places.
Here’s what you need to do:
- A view (i.e. your custom keyboard nib) needs to be of a class that
implements the
UIInputViewAudioFeedback
protocol. - The class should implement the
enableInputClicksWhenVisible
method to return YES. - The class can then call
[[UIDevice currentDevice] playInputClick]
whenever it likes.
Have a look at NerdKeyboard.xib, NerdKeyboardView.m, and NerdKeyboardView.h for the gory details.
You can always customize the sound playback too but using playInputClick
means you don’t have to worry about having an audio file or checking the
system volume/mute switch.
In Closing
Of course, real programmers only need zeros…